PHP AES加密
示例
<?php
/**
* User: orzblankcat
* Date: 2019/1/25
* Time: 17:30
*/
class AesClass
{
#电子密码本模式(Electronic Codebook, ECB)
#密码分组链接模式(Cipher Block Chaining, CBC)
#计算器模式(Counter, CTR)
#密码反馈模式(Cipher Feedback, CFB)
#输出反馈模式(Output Feedback, OFB)和XTS 加密(XEX Tweak + Ciphertext Stealing)模式。
#CCM (counter with CBC-MAC)定义在分组长度为128位的加密算法中,如,AES 的分组长度为128。组成AES-CCM算法的关键组成是CTR工作模式以及CMAC认证算法。
#GCM基于并行化设计,因此可以提供高效的吞吐率和低成本、低时延。本质是消息在变形的CTR模式下加密,密文结果与密钥以及消息长度在GF(2^128)域上相乘。其输入输出和CCM基本一致。
#GCM中的G就是指GMAC,C就是指CTR。GCM可以提供对消息的加密和完整性校验,另外,它还可以提供附加消息的完整性校验。在实际应用场景中,有些信息是我们不需要保密,但信息的接收者需要确认它的真实性的,例如源IP,源端口,目的IP,IV,等等。因此,我们可以将这一部分作为附加消息加入到MAC值的计算当中。最后,密文接收者会收到密文、IV(计数器CTR的初始值)、MAC值。
protected $method = "BF-CBC";
protected $password = "123456";
protected $options = OPENSSL_RAW_DATA;
protected $tag;// 使用 AEAD 密码模式(GCM 或 CCM)时传引用的验证标签 php7.1以上生效
protected $aad = "";//php7.1以上生效
protected $tag_length = 16;//php7.1以上生效
protected $iv;
public function __construct()
{
extension_loaded('openssl') or die('php需要openssl扩展支持');
#获取密码初始化向量(iv)长度
$iv_length = openssl_cipher_iv_length($this->method);
#生成一个伪随机字节串
$this->iv = openssl_random_pseudo_bytes($iv_length);
}
/**
* 获取所有支持的加密模式
*/
public function getMethods()
{
var_dump(openssl_get_cipher_methods());
}
/**
* 算法加密
* @param $data string 数据
* @return string
*/
public function encryptKey($data)
{
if (PHP_VERSION_ID >= 70100 && mb_stristr($this->method,"GCM") || PHP_VERSION_ID >= 70100 && mb_stristr($this->method,"CCM"))
{
$decrypted = openssl_encrypt($data,$this->method,$this->password,$this->options,$this->iv,$this->tag,$this->aad,$this->tag_length);
}else
{
$decrypted = openssl_encrypt($data,$this->method,$this->password,$this->options,$this->iv);
}
return base64_encode($decrypted);
}
/**
* 算法解密
* @param $data string 数据
* @return string
*/
public function decodeKey($data)
{
$encrypted = base64_decode($data);
if (PHP_VERSION_ID >= 70100 && mb_stristr($this->method,"GCM") || PHP_VERSION_ID >= 70100 && mb_stristr($this->method,"CCM"))
{
return openssl_decrypt($encrypted, $this->method, $this->password,$this->options,$this->iv,$this->tag,$this->aad);
}else
{
return openssl_decrypt($encrypted, $this->method, $this->password,$this->options,$this->iv);
}
}
}
<?php
/**
* User: orzblankcat
* Date: 2019/1/25
* Time: 17:30
*/
class AesClass
{
#电子密码本模式(Electronic Codebook, ECB)
#密码分组链接模式(Cipher Block Chaining, CBC)
#计算器模式(Counter, CTR)
#密码反馈模式(Cipher Feedback, CFB)
#输出反馈模式(Output Feedback, OFB)和XTS 加密(XEX Tweak + Ciphertext Stealing)模式。
#CCM (counter with CBC-MAC)定义在分组长度为128位的加密算法中,如,AES 的分组长度为128。组成AES-CCM算法的关键组成是CTR工作模式以及CMAC认证算法。
#GCM基于并行化设计,因此可以提供高效的吞吐率和低成本、低时延。本质是消息在变形的CTR模式下加密,密文结果与密钥以及消息长度在GF(2^128)域上相乘。其输入输出和CCM基本一致。
#GCM中的G就是指GMAC,C就是指CTR。GCM可以提供对消息的加密和完整性校验,另外,它还可以提供附加消息的完整性校验。在实际应用场景中,有些信息是我们不需要保密,但信息的接收者需要确认它的真实性的,例如源IP,源端口,目的IP,IV,等等。因此,我们可以将这一部分作为附加消息加入到MAC值的计算当中。最后,密文接收者会收到密文、IV(计数器CTR的初始值)、MAC值。
protected $method = "BF-CBC";
protected $password = "123456";
protected $options = OPENSSL_RAW_DATA;
protected $tag;// 使用 AEAD 密码模式(GCM 或 CCM)时传引用的验证标签 php7.1以上生效
protected $aad = "";//php7.1以上生效
protected $tag_length = 16;//php7.1以上生效
protected $iv;
public function __construct()
{
extension_loaded('openssl') or die('php需要openssl扩展支持');
#获取密码初始化向量(iv)长度
$iv_length = openssl_cipher_iv_length($this->method);
#生成一个伪随机字节串
$this->iv = openssl_random_pseudo_bytes($iv_length);
}
/**
* 获取所有支持的加密模式
*/
public function getMethods()
{
var_dump(openssl_get_cipher_methods());
}
/**
* 算法加密
* @param $data string 数据
* @return string
*/
public function encryptKey($data)
{
if (PHP_VERSION_ID >= 70100 && mb_stristr($this->method,"GCM") || PHP_VERSION_ID >= 70100 && mb_stristr($this->method,"CCM"))
{
$decrypted = openssl_encrypt($data,$this->method,$this->password,$this->options,$this->iv,$this->tag,$this->aad,$this->tag_length);
}else
{
$decrypted = openssl_encrypt($data,$this->method,$this->password,$this->options,$this->iv);
}
return base64_encode($decrypted);
}
/**
* 算法解密
* @param $data string 数据
* @return string
*/
public function decodeKey($data)
{
$encrypted = base64_decode($data);
if (PHP_VERSION_ID >= 70100 && mb_stristr($this->method,"GCM") || PHP_VERSION_ID >= 70100 && mb_stristr($this->method,"CCM"))
{
return openssl_decrypt($encrypted, $this->method, $this->password,$this->options,$this->iv,$this->tag,$this->aad);
}else
{
return openssl_decrypt($encrypted, $this->method, $this->password,$this->options,$this->iv);
}
}
}
<?php /** * User: orzblankcat * Date: 2019/1/25 * Time: 17:30 */ class AesClass { #电子密码本模式(Electronic Codebook, ECB) #密码分组链接模式(Cipher Block Chaining, CBC) #计算器模式(Counter, CTR) #密码反馈模式(Cipher Feedback, CFB) #输出反馈模式(Output Feedback, OFB)和XTS 加密(XEX Tweak + Ciphertext Stealing)模式。 #CCM (counter with CBC-MAC)定义在分组长度为128位的加密算法中,如,AES 的分组长度为128。组成AES-CCM算法的关键组成是CTR工作模式以及CMAC认证算法。 #GCM基于并行化设计,因此可以提供高效的吞吐率和低成本、低时延。本质是消息在变形的CTR模式下加密,密文结果与密钥以及消息长度在GF(2^128)域上相乘。其输入输出和CCM基本一致。 #GCM中的G就是指GMAC,C就是指CTR。GCM可以提供对消息的加密和完整性校验,另外,它还可以提供附加消息的完整性校验。在实际应用场景中,有些信息是我们不需要保密,但信息的接收者需要确认它的真实性的,例如源IP,源端口,目的IP,IV,等等。因此,我们可以将这一部分作为附加消息加入到MAC值的计算当中。最后,密文接收者会收到密文、IV(计数器CTR的初始值)、MAC值。 protected $method = "BF-CBC"; protected $password = "123456"; protected $options = OPENSSL_RAW_DATA; protected $tag;// 使用 AEAD 密码模式(GCM 或 CCM)时传引用的验证标签 php7.1以上生效 protected $aad = "";//php7.1以上生效 protected $tag_length = 16;//php7.1以上生效 protected $iv; public function __construct() { extension_loaded('openssl') or die('php需要openssl扩展支持'); #获取密码初始化向量(iv)长度 $iv_length = openssl_cipher_iv_length($this->method); #生成一个伪随机字节串 $this->iv = openssl_random_pseudo_bytes($iv_length); } /** * 获取所有支持的加密模式 */ public function getMethods() { var_dump(openssl_get_cipher_methods()); } /** * 算法加密 * @param $data string 数据 * @return string */ public function encryptKey($data) { if (PHP_VERSION_ID >= 70100 && mb_stristr($this->method,"GCM") || PHP_VERSION_ID >= 70100 && mb_stristr($this->method,"CCM")) { $decrypted = openssl_encrypt($data,$this->method,$this->password,$this->options,$this->iv,$this->tag,$this->aad,$this->tag_length); }else { $decrypted = openssl_encrypt($data,$this->method,$this->password,$this->options,$this->iv); } return base64_encode($decrypted); } /** * 算法解密 * @param $data string 数据 * @return string */ public function decodeKey($data) { $encrypted = base64_decode($data); if (PHP_VERSION_ID >= 70100 && mb_stristr($this->method,"GCM") || PHP_VERSION_ID >= 70100 && mb_stristr($this->method,"CCM")) { return openssl_decrypt($encrypted, $this->method, $this->password,$this->options,$this->iv,$this->tag,$this->aad); }else { return openssl_decrypt($encrypted, $this->method, $this->password,$this->options,$this->iv); } } }
备注:还未亲自测试,仅供参考
版权声明:
作者:admin
链接:http://blog.mryxh.cn/2679.html
文章版权归作者所有,未经允许请勿转载。
THE END

0
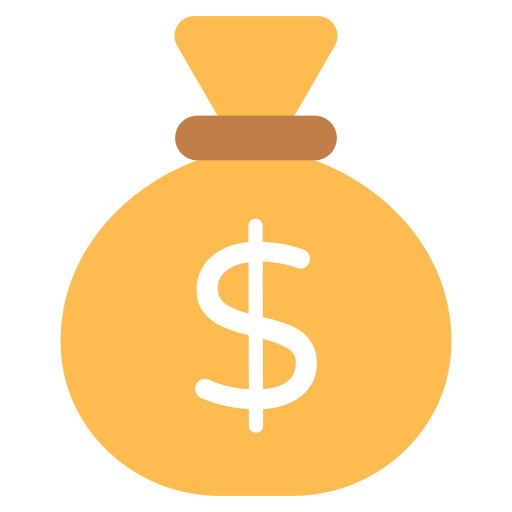
打赏

分享

二维码
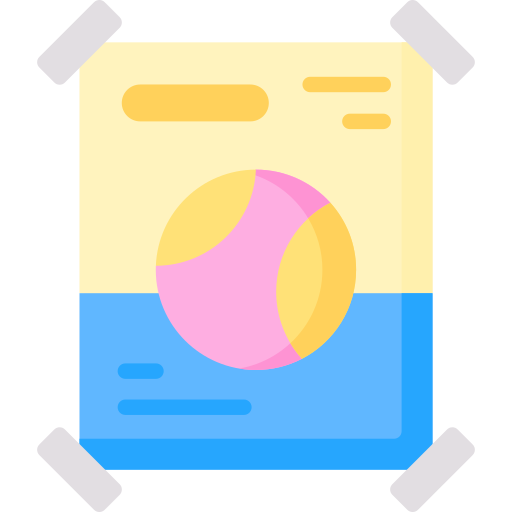
海报